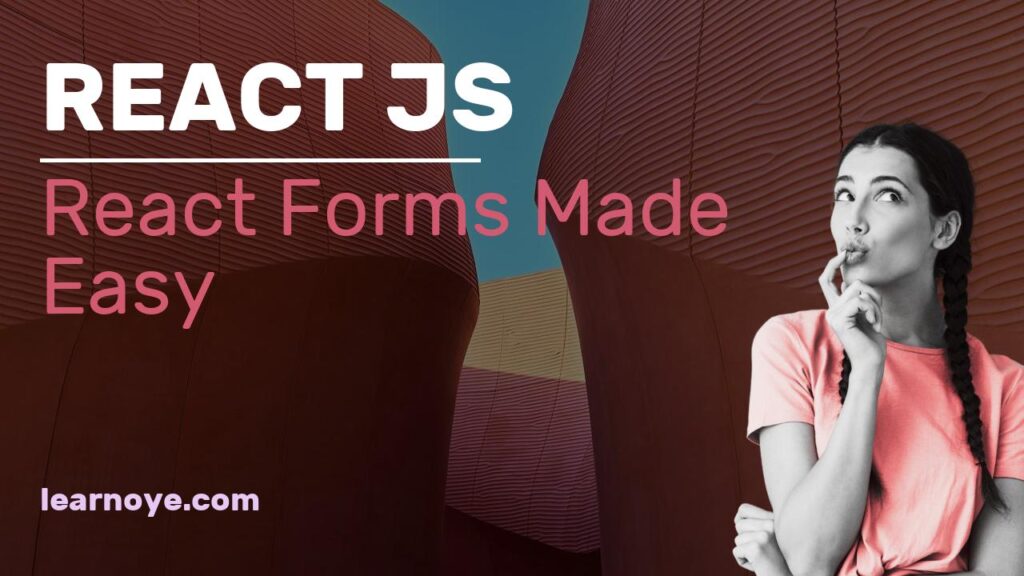
React Hooks core concepts
React Hooks are functions that let you “hook into” React state and lifecycle features from function components. They were introduced in React 16.8 and provide a more concise and expressive way to manage state, side effects, and other React features without writing class components.
Here are the core concepts of React Hooks:
1. State Hook (useState
)
- Purpose: Allows functional components to have state, similar to
this.state
in class components. - Usage:
const [state, setState] = useState(initialState);
state
: The current state value.setState
: A function to update the state. Unlikethis.setState
in classes, it doesn’t merge the old state with the new one; it replaces it. You can pass a function tosetState
to update the state based on the previous state if necessary.
- Example:
import React, { useState } from "react";
function Counter() {
const [count, setCount] = useState(0);
return (
<div>
<p>Count: {count}</p>
<button onClick={() => setCount(count + 1)}>Increment</button>
<button onClick={() => setCount((prevCount) => prevCount - 1)}>
Decrement
</button>
</div>
);
}
2. Effect Hook (useEffect
)
- Purpose: Performs side effects (e.g., data fetching, DOM manipulation, subscriptions) in functional components. It’s a combination of
componentDidMount
,componentDidUpdate
, andcomponentWillUnmount
from class components. - Usage:
useEffect(effectFunction, dependencyArray);
effectFunction
: The function containing the side effect logic.dependencyArray
: An optional array of values. If provided, the effect will only re-run when one of the values in the array changes. An empty array ([]
) means the effect runs only once after the initial render (likecomponentDidMount
). Omitting the array makes the effect run after every render.
- Cleanup Function: The
effectFunction
can optionally return a cleanup function. This function is executed before the effect runs again and when the component unmounts (likecomponentWillUnmount
). This is essential for preventing memory leaks and unwanted behavior. - Example:
import React, { useState, useEffect } from "react";
function DataFetcher() {
const [data, setData] = useState(null);
useEffect(() => {
const fetchData = async () => {
const response = await fetch("https://api.example.com/data");
const jsonData = await response.json();
setData(jsonData);
};
fetchData();
return () => {
// Cleanup function (optional)
// Abort any ongoing fetch requests, etc.
};
}, []); // Empty dependency array - runs only once
return (
<div>
{data ? <pre>{JSON.stringify(data, null, 2)}</pre> : <p>Loading...</p>}
</div>
);
}
3. Custom Hooks
- Purpose: Abstract reusable logic into custom functions. These functions can use other hooks internally.
- Naming: Custom hooks must start with
use
. - Example:
import { useState, useEffect } from "react";
function useWindowWidth() {
const [width, setWidth] = useState(window.innerWidth);
useEffect(() => {
const handleResize = () => setWidth(window.innerWidth);
window.addEventListener("resize", handleResize);
return () => window.removeEventListener("resize", handleResize); // Cleanup
}, []);
return width;
}
function MyComponent() {
const width = useWindowWidth();
return <p>Window width: {width}</p>;
}
Rules of Hooks:
- Only Call Hooks at the Top Level: Don’t call Hooks inside loops, conditions, or nested functions.
- Only Call Hooks from React Functions: Call them from functional components or custom Hooks. Don’t call Hooks from regular JavaScript functions.
These are the foundational concepts of React Hooks. There are additional built-in Hooks like useContext
, useReducer
, useCallback
, useMemo
, useRef
, and more, each serving a specific purpose to enhance the functionality and organization of your React components. Understanding these core concepts is key to effectively using Hooks in your projects.
Leave a Reply